To integrate our web push notifications tool on your page you need:
- an indigitall account
- access to your Google Tag Manager account (GTM)
Integration
-
Once you are logged into your GTM account, click on Add new tag.
-
Choose a title (eg indigitall) and hit the pencil icon under Tag Settings .
-
On the next screen, under Custom, you must choose Custom HTML. Now paste the following code snippet:
The appKey value is unique and personal. You can get it by accessing your account> settings> App Key value
<script>
(function () {
var el = document.createElement('script');
el.src = '/sdk.min.js';
el.async = 'true';
el.addEventListener('load', function () {
indigitall.init({
appKey: 'yourPersonalAppKey',
workerPath: '/worker.min.js'
});
});
document.head.appendChild(el);
})();
</script>
- Scrolling down, you will see the term: activation and the icon of a pencil that you will have to click:
- In Choose an activator select All Pages and press add
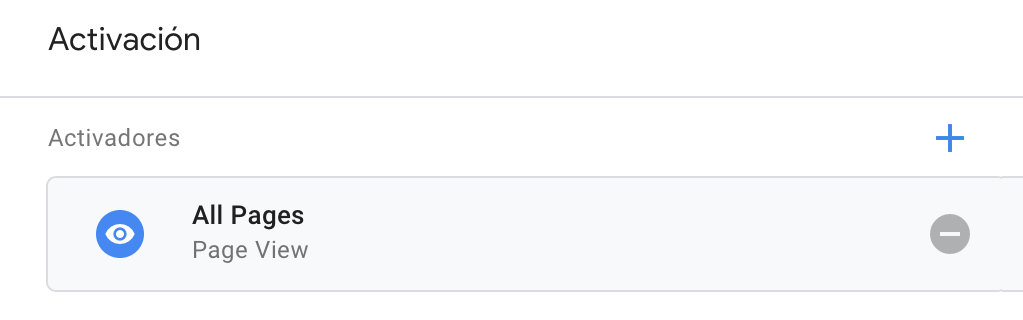
- Click on the Save button.
- Download two files and upload them to the main folder of your website.
How to upload files to your server or access them?
You can do it through FTP or from your server's control panel (cPanel, directAdmin, etc) among other ways. Below you will find an explanatory video of the process. In less than 10 minutes, you should have it.
You can ask your hosting provider or your trusted programmer for help by sending these instructions.
Check the integration
If everything went well, when you access your website again, you should see a message in your browser to allow notifications. Example:
Click Allow.
Then open the developer console (hit F12) and go to Console, you should see something like this:
[IND] Client: Method: PUT
URL: https://device-api.indigitall.com/v1/device?appKey=(yourAppKey)&deviceId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
Request Body: {
[ ]
"pushToken": "https://fcm.googleapis.com/fcm/send/xxxx",s
"browserPublicKey": "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
"browserPrivateKey": "xxxxxxxxxxxx"
}
Response Code: 200
Response Message: OK
Response Body: {
"deviceId": "xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx",
"enabled": true,
"platform": "webpush"
}
Note that Response Code has the value 200.
If everything is correct, congratulations, you just need to create and send your first campaign!
Some WordPress optimization plugins as Autoptimize block our plugin execution.
If you have this problem, add the folder "wp-content/plugins/indigitall-web-push-notifications" to your exception list.
Advaced Guide
Devices
This section describes the different actions that could be done on a device registered in indigitall. The device model has the following structure:
device = {
deviceId: "string",
pushToken: "string",
browserPublicKey: "string",
browserPrivateKey: "string",
platform: "string",
version: "string",
productName: "string",
productVersion: "string",
browserName: "string",
browserVersion: "string",
osName: "string",
osVersion: "string",
deviceType: "string",
enabled: "boolean"
};
- Get the device
To get the device you have to make the call that can be seen in the following code. If the operation was successful, the DeviceCallback method returns a Device object.
<script>
indigitall.deviceGet((device) => {
// success function
console.log(device);
},() => {
// error function
});
</script>
- Activate the device
The application is able to manage the current device status on the indigitall platform. It is possible to activate this device from the application itself. With the method that we can see below we would be able to return the Device object if the operation has been carried out satisfactorily.
<script>
indigitall.deviceEnable((device) => {
// success function
console.log(device);
},() => {
// error function
});
</script>
- Deactivate the device
As we have seen in the previous point, it is possible to deactivate the device from the application itself. To do this, you have to execute the code that we can see below. If the operation has been carried out successfully, the callback with the name deviceDisable will return a Device object.
<script>
indigitall.deviceDisable((device) => {
// success function
console.log(device);
},() => {
// error function
});
</script>
- Use cases
This example code creates a section on your website where the status of the device is checked (being active or inactive).
<div id="notifications-manager">
<p>Notifications:</p>
<!-- Rounded switch -->
<label class="switch">
<input type="checkbox">
<span class="slider round" style=""></span>
</label>
</div>
<script>
$(() => {
indigitall.deviceGet((device) => {
if (device && device.enabled === true) {
$('.switch input[type=checkbox]')[0].checked = true;
} else {
$('.switch input[type=checkbox]')[0].checked = false;
}
});
$('.switch span.slider').click(() => {
if ($('.switch input[type=checkbox]')[0].checked === true) {
indigitall.deviceDisable((device) => {
console.log ('device disabled');
})
} else {
indigitall.deviceEnable((device) => {
console.log ('device enabled');
})
}
0});
});
</script>
Topics
This section describes the operations available on the themes. The Topic object has the following structure:
topics = [{
code: "string",
name: "string",
subscribed: "boolean",
visible: "boolean",
parentCode: "string"
},
{
}];
- List of Topics
Thanks to the following method we can get a list of topics. This method will return a TopicsCallback, in charge of returning an array of Topic objects if the operation is successful.
<script>
indigitall.topicsList((topics) => {
// success function
console.log(topics);
}, () => {
// error function
});
</script>
- Subscription
The current device may be subscribed to multiple channels. The following method returns an array of Topic objects if the operation has been successful.
<script>
// Remember to replace with your topicCodes
var topicsCodes = ["001", ];
indigitall.topicsSubscribe(topicsCodes, (topics) => {
// success function
console.log(topics);
}, () => {
// error function
});
</script>
- Unsubscribe
As the current device could be subscribed to several channels, it could be unsubscribed from these channels. The subsequent code shows us how an array of Topic objects is returned if the operation is successful.
<script>
// Remember to replace with your topicCodes
var topicCodes = ["001", ];
indigitall.topicsUnsubscribe(topicCodes, (topics) => {
// success function
console.log(topics);
}, () => {
// error function
});
</script>
- Use cases
First of all you have to create the topics (see [# PlatformManual / Filters / TargetGroups] (/en/ platformmanual / filters / targetgroups.html)) through the [ indigitall ] console(https://console.indigitall.com).
In this example we use a theme with the name: Insurance and the code: 001.
- Insurance -> 001
How to subscribe and unsubscribe topics
In this case we will use the previous example as a base to extend it with the code that we can see below.
<div id="notifications-manager">
<p>Topics:</p>
<ul class="topics" style="list-style: none">
</ul>
</div>
<script>
$(() => {
indigitall.topicsList((topics) => {
topics.forEach((topic) => {
$("ul.topics").append(`<li><input type="checkbox"
id="${topic.code}"
${topic.subscribed ? 'checked' : ''}/>
${topic.name}</li>`)
});
$('ul.topics li input[type="checkbox"]').click((e) => {
if (e.target.checked === true) {
indigitall.topicsSubscribe([e.target.id]);
} else {
indigitall.topicsUnsubscribe([e.target.id])
}
})
});
});
</script>
- Subscribing to a topic after a while
In this case we are going to subscribe to a topic after 10 seconds have passed (which is 10,000 milliseconds, as we see in the code).
<script>
setTimeout(() => {
indigitall.topicsSubscribe(['001']);
}, 10000);
</script>
- Subscribe to a topic when an event occurs
The code below represents the way to subscribe to a topic when an event occurs.
<script>
document.addEventListener('myEvent', () => {
indigitall.topicsSubscribe(['001']);
});
</script>